Post by curious48 on Jul 11, 2016 0:44:44 GMT
In this post I wanted to summarize the experience of moving from a PC (including small embedded PC's such as the Raspberry Pi) to microcontrollers and electronics such as Arduino, if you've only ever worked with PC's. Especially if you've already played around with learning Linux and some more advanced things.
Some of this also applies to people who are learning both for the first time.
Firstly, why would you move into using Arduino or other microcontrollers?
Well, when it comes to controlling the real world, we live in an analog world. Doors open and close with a hinge. Wipers wipe, scrubbers scrub, lifters lift, pulleys pull, and none of this is something you can "apt-get" or download as a Windows or Mac executable.
The real world doesn't answer a ping or a bluetooth connection, or even GPIO pins. You can't telnet or SSH into your hair drier, if you need a source of hot heat for your aquarium. Even the thermometer you use to decide to do that doesn't have a configuration file. It doesn't take USB. It doesn't take bluetooth. And it won't communicate over serial or GPIO. It's all analog.
Likewise, if you try to build and fly a custom quadcopter with your own four motors, those motors might not cost much - but that red and black wire going to the motor doesn't take Ethernet. So if you're really building something from scratch - if you're really controlling the world, there is much that an embedded PC stops short of. A Raspberry Pi is very smart: the $5 version can do a billion operations per second, along with its GPU more like 50 billion. But without a great deal of real-world circuitry, it can't so much as open a door. They're just computing. All of the Linux configuration in the world can't help you do anything in the real world, without a lot of additional tools.
This is a huge transition for people who have only worked on PC's, mostly due to the type of knowledge required and skills involved. Even experts on PC's don't necessarily know anything about circuits or microcontrollers. You could take a software engineer who runs Google's servers, serving millions of pages with fail-safe redundancy, logging, and extremely complicated setups and distributed databases. This engineer could have 15 years of experience. Still, if asked how many volts are running through the CPU in their own - or any other - CPU, they might never have thought about it. The world of PC's for users is very far removed from the analog and low-level electronics world. You do not even have to understand "W=AV" (watts = amps * volts, remember it as "wave") in order to be an expert PC user who can do anything on any computer. Computers have interfaces that you plug and unplug and understand at a very high level. They don't require reading circuit diagrams to use.
Low-level electronics are different. What are some of the differences?
Firstly, circuits. When you see a circuit diagram for the first time, you won't understand it. It's not easy. There's a good chance that if you copy down or use the wrong resistors, capacitors, and so forth, you will actually release the smoke from the poor little motor you just overloaded. This doesn't happen when you plug and unplug USB devices.
Secondly, you will not wire things up correctly. 85% of the actual, correct circuits you create, you will wire up, and the first time you turn it on, it simply won't work as you've just wired it up. The reason could be something as simple as not inserting wires all the way. Or not getting the circuit right. But it simply does not work. When you join two wires, they might come apart later. This doesn't happen on the PC - when you write a configuration file, it stays written.
Next, a small intermission.
Okay, end of Intermission.
------
So, this is the same thing with electronics. While on the PC you can work with a ton of languages, (not just Java, Ruby, Go, Python, PHP, Javascript or Node.js, bash scripting, the list goes on and on... and yes R, too!) - the PC is not going to drive your analog electronic circuits. A microprocessor will do that.
And the language you use to program microprocessors is very close to C (basically is C) - including the Arduino language. An advanced user might use R (a statistical language) to analyze your sensor readings later on a PC, but when it comes to actually collecting them using a microcontroller, you must learn to love C.
C is a low-level language. It is very close to the actual registers, the actual instructions that the microprocessor will be executing. There is not a lot of overhead. Although some libraries, increase how much can be done, they are very far from the large programs that are run on PC's, or the Raspberry Pi. There is also several orders of magnitude difference in the amount of computing available.
That means that when you transition from the PC to programming a circuit with a microcontroller, you have to throw out all of the experience you have installing applications, writing scripts and configuration files, using complicated frameworks or modules, and get down to actual C.
An example of a common task programming a PC versus programming a microcontroller:
PC programming:
Since this post is aimed at people coming from a background on the PC, here is what a typical small program looks like on a PC. I simply googled an example. In this case this program is a shell script that pings Google, I've stopped here and just print out the results:
These few lines of shell script already do so much: it fires up a network program (ping) and goes out over the Internet to Google's servers, measuring the result. In a microcontroller-project, even including Ethernet functionality would be a huge extra step.
As a next step, instead of pinging Google, I could ping one of my servers. Finally, I could automatically download a file, or use a so-called "regular expression" to get something of interest out of it.
If the ping times don't look good, or if the server is down, I could use a single line to send myself mail using a gmail account [edit: this particular solution doesn't work well at the moment], to notify me that my server is unavailable. The whole program could still end up being just a few lines. While I'm not going to go ahead and make the complete example, let's look at everything that is happening here: automatically pinging a server; or I could have used wget/curl to download page contents; easily possible to parse using a regular expression in just a line or two (not shown); I could send mail using sendmail. Likewise, complex software exists that does things like record video and sound, do machine vision, or any number of extremely extensive, high-level tasks.
All of this is easy, very high-level, PC stuff. If I didn't want to write it myself, I could download a graphical application and do it. The Raspberry Pi even has a desktop interface, you can boot it up and use it like a PC. Relatively easily, if someone else has done it.
Microcontroller programming
Now let's look at a typical program in Arduino for reading a "10k ohm potentiometer", an extremely simple task that is typical of what you might be doing:
That's it. On the one hand, it works at an extremely low level, as compared with running desktop applications, connecting to a database, saving a video file, or any number of other tasks. On the other, it is not making use of any complicated programs, frameworks, or languages. The sendmail link I posted that can send to gmail goes through many, many more low-level functions than what I've just quoted. So does Python or any other scripting language you use. The Arduino code is extremely simple, but works line by line at a low level, or uses libraries that are a few KB in size. An installation of just a database like MySQL might be 80+ megabytes on the Raspberry Pi, while the entire memory of an Arduino-compatible board might be 8KB (in the case of the Arduino-compatible Attiny85). That is a difference in size of 10000x (80MB vs 8 KB). You can see someone bump into this wall, writing just a few low-level LED commands.
There are some libraries that enable additional functionality, but the speed of a microcontroller is limited to a few megahertz, it has a limited amount of memory, and it doesn't run a complete operating system like Linux, Windows or a Mac. So why do people use it?
But look at this line, from the above code example:
* Analog sensor (potentiometer will do) attached to analog input 0
That's the magic line. The interface with the real world. Here's how the circuit looks powered up:
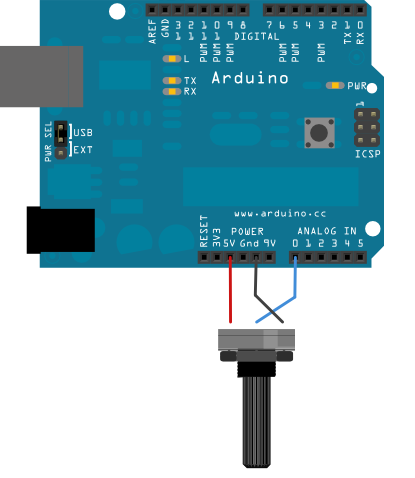
And there is a lot of potential to get things wrong while you do that. But if you don't get it wrong - now you're reading a potentiometer, which is essentially an analog knob you can turn:
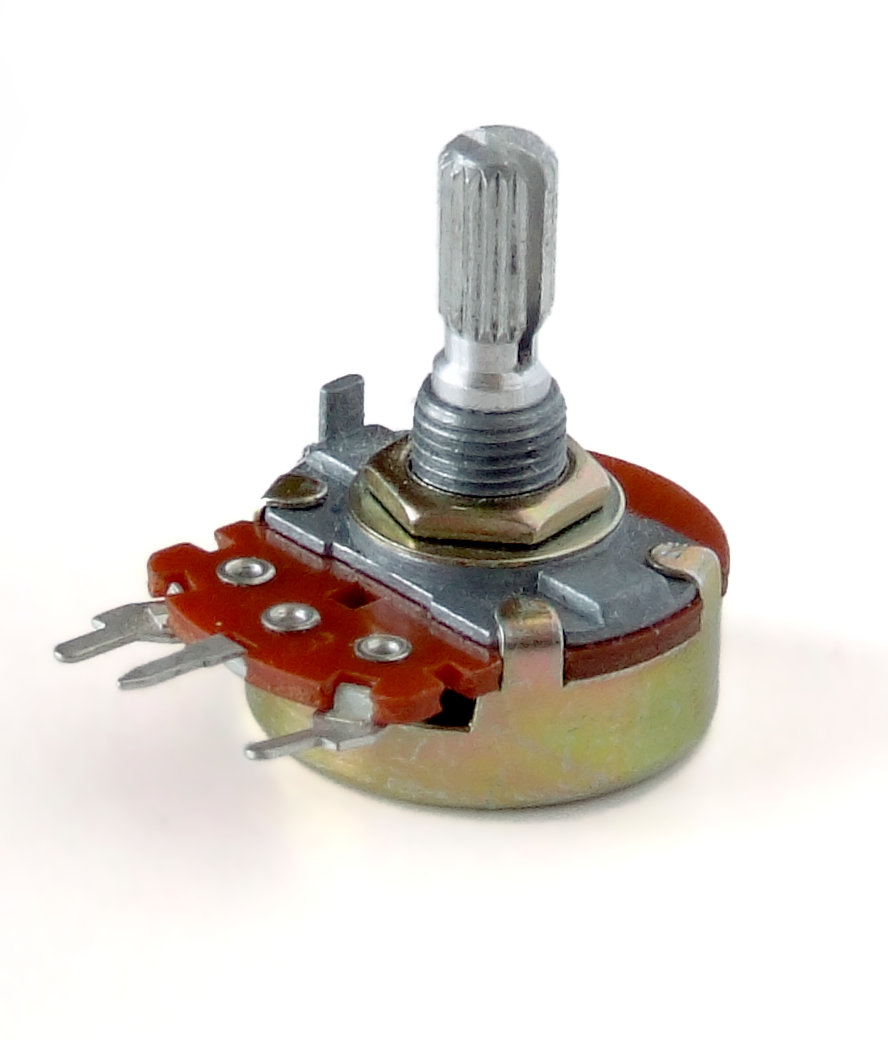
A 10k ohm potentiometer costs $0.25 (okay, up to a dollar or two in a quantity of one). For pennies, you can build an analog dial to your specifications, read it, and drive some motor based on the results. But you will have to come up with the circuit diagram. Which requires a relatively deep level of understanding. For example, why 10k ohm? What even is an ohm? You have to learn these things, get the components, experiment and copy circuits.
to wire up components in circuits, getting resulst that look like this:

to get servos and motors that look like this:

and eventually running robots that look like this:

On the PC side, without analog inputs, how would you even get past the very first step - reading a single potentiometer or analog input? I suppose you could find some kind of ready-made dial that interfaces over USB. But this will neve scale or introduce anyone to electronics.
Arduino, and microcontrollers, is about learning and using the things that are interfacing with the real world, from garage openers and thermometer readers, to complex robotics. Of course, just as in the last picture the robot is certainly interfacing with a PC or computer driving it, it is likely that at some point you might again circle back to knowledge of Linux and a PC. But only after you have built and used the circuits that power devices that interact with the real world.
Summary
So the entire nature of the whole endeavor is completely different. I found that moving into the microcontroller things was a steep learning curve, particularly due to the need to wire things up. It's not "plug and play." You have to figure out what's going on, read, and follow new kinds of instructions, specifically, breadboards, components, circuit diagrams. Luckily, the Arduino community is an extremely good resource for getting started.
So, that about sums up what you can expect when moving into Arduino-type electronics from PC's:
1) You will be able to do real-world things, actually build something that moves or interfaces with the analog world we live in. It's not plug and play USB interfaces anymore.
2) You have to learn and understand completely new things, specifically circuits, and programming a Microcontroller in Arduino or another low-level language.
3) Nothing will work the first time. You might destroy some small components.
4) The hardware used are different. You will need a microcontroller, which speaks a low level language like C, called Arduino, or you can program microcontrollers directly in a similar way, and flash these from a PC. You will also be using electronics components.
Eventually, if you want, you can move to programming the same microcontrollers that are used in real-world electronics devices.
At the end of all this, the reward should certainly be worth it. But it is a big transition, to be sure. I hope you've found this post helpful in reviewing some of the things you might expect moving from PC's to Arduino-type Electronics. Of course, electronics goes very deep! After all, even within a PC someone had to design every single resistor or transistor that is on the motherboard of your computer, which would have a circuit diagram that runs into hundreds of thousands of pages. Including the billion transistors in your CPU itself. While you never have to go that far - just as, as a PC user, you will not have to dive into Kernel code on the programming side - the whole nature of working with electronics is different from working with a PC.
At times, it's frustrating, but also a lot of fun. Just don't expect anything to work the first time. Even if you've copied and pasted it.
Welcome to the world of microcontrollers and electronics, and I hope you will learn a lot of new things.
Some of this also applies to people who are learning both for the first time.
Firstly, why would you move into using Arduino or other microcontrollers?
Well, when it comes to controlling the real world, we live in an analog world. Doors open and close with a hinge. Wipers wipe, scrubbers scrub, lifters lift, pulleys pull, and none of this is something you can "apt-get" or download as a Windows or Mac executable.
The real world doesn't answer a ping or a bluetooth connection, or even GPIO pins. You can't telnet or SSH into your hair drier, if you need a source of hot heat for your aquarium. Even the thermometer you use to decide to do that doesn't have a configuration file. It doesn't take USB. It doesn't take bluetooth. And it won't communicate over serial or GPIO. It's all analog.
Likewise, if you try to build and fly a custom quadcopter with your own four motors, those motors might not cost much - but that red and black wire going to the motor doesn't take Ethernet. So if you're really building something from scratch - if you're really controlling the world, there is much that an embedded PC stops short of. A Raspberry Pi is very smart: the $5 version can do a billion operations per second, along with its GPU more like 50 billion. But without a great deal of real-world circuitry, it can't so much as open a door. They're just computing. All of the Linux configuration in the world can't help you do anything in the real world, without a lot of additional tools.
This is a huge transition for people who have only worked on PC's, mostly due to the type of knowledge required and skills involved. Even experts on PC's don't necessarily know anything about circuits or microcontrollers. You could take a software engineer who runs Google's servers, serving millions of pages with fail-safe redundancy, logging, and extremely complicated setups and distributed databases. This engineer could have 15 years of experience. Still, if asked how many volts are running through the CPU in their own - or any other - CPU, they might never have thought about it. The world of PC's for users is very far removed from the analog and low-level electronics world. You do not even have to understand "W=AV" (watts = amps * volts, remember it as "wave") in order to be an expert PC user who can do anything on any computer. Computers have interfaces that you plug and unplug and understand at a very high level. They don't require reading circuit diagrams to use.
Low-level electronics are different. What are some of the differences?
Firstly, circuits. When you see a circuit diagram for the first time, you won't understand it. It's not easy. There's a good chance that if you copy down or use the wrong resistors, capacitors, and so forth, you will actually release the smoke from the poor little motor you just overloaded. This doesn't happen when you plug and unplug USB devices.
Secondly, you will not wire things up correctly. 85% of the actual, correct circuits you create, you will wire up, and the first time you turn it on, it simply won't work as you've just wired it up. The reason could be something as simple as not inserting wires all the way. Or not getting the circuit right. But it simply does not work. When you join two wires, they might come apart later. This doesn't happen on the PC - when you write a configuration file, it stays written.
Next, a small intermission.
I'd like to tell a Pirate joke. Go ahead and read it out loud now, and then tell it to your friends the same way:
(you) "Let me tell you a pirate joke."
(your friend) "Okay..."
"What's a Pirate's favorite letter?"
(your friend, guessing:) "Arrr?"
"No, no, a Pirate's first love will always be the 'c'."
(If instead, they say, "I don't know, what" then you can say "Well, a lot of people think it's Arrr.... - your friend might laugh - but a Pirate's first love will always be the 'C'....")
(you) "Let me tell you a pirate joke."
(your friend) "Okay..."
"What's a Pirate's favorite letter?"
(your friend, guessing:) "Arrr?"
"No, no, a Pirate's first love will always be the 'c'."
(If instead, they say, "I don't know, what" then you can say "Well, a lot of people think it's Arrr.... - your friend might laugh - but a Pirate's first love will always be the 'C'....")
Okay, end of Intermission.
------
So, this is the same thing with electronics. While on the PC you can work with a ton of languages, (not just Java, Ruby, Go, Python, PHP, Javascript or Node.js, bash scripting, the list goes on and on... and yes R, too!) - the PC is not going to drive your analog electronic circuits. A microprocessor will do that.
And the language you use to program microprocessors is very close to C (basically is C) - including the Arduino language. An advanced user might use R (a statistical language) to analyze your sensor readings later on a PC, but when it comes to actually collecting them using a microcontroller, you must learn to love C.
C is a low-level language. It is very close to the actual registers, the actual instructions that the microprocessor will be executing. There is not a lot of overhead. Although some libraries, increase how much can be done, they are very far from the large programs that are run on PC's, or the Raspberry Pi. There is also several orders of magnitude difference in the amount of computing available.
That means that when you transition from the PC to programming a circuit with a microcontroller, you have to throw out all of the experience you have installing applications, writing scripts and configuration files, using complicated frameworks or modules, and get down to actual C.
An example of a common task programming a PC versus programming a microcontroller:
PC programming:
Since this post is aimed at people coming from a background on the PC, here is what a typical small program looks like on a PC. I simply googled an example. In this case this program is a shell script that pings Google, I've stopped here and just print out the results:
#!/bin/bash
#ping google.com and store the results into the variable results
#while redirecting STEDERR to there too (to silence errors)
results=$(ping -c 4 -q google.com 2>&1);
#just print the results for now
echo $results;
exit;
These few lines of shell script already do so much: it fires up a network program (ping) and goes out over the Internet to Google's servers, measuring the result. In a microcontroller-project, even including Ethernet functionality would be a huge extra step.
As a next step, instead of pinging Google, I could ping one of my servers. Finally, I could automatically download a file, or use a so-called "regular expression" to get something of interest out of it.
If the ping times don't look good, or if the server is down, I could use a single line to send myself mail using a gmail account [edit: this particular solution doesn't work well at the moment], to notify me that my server is unavailable. The whole program could still end up being just a few lines. While I'm not going to go ahead and make the complete example, let's look at everything that is happening here: automatically pinging a server; or I could have used wget/curl to download page contents; easily possible to parse using a regular expression in just a line or two (not shown); I could send mail using sendmail. Likewise, complex software exists that does things like record video and sound, do machine vision, or any number of extremely extensive, high-level tasks.
All of this is easy, very high-level, PC stuff. If I didn't want to write it myself, I could download a graphical application and do it. The Raspberry Pi even has a desktop interface, you can boot it up and use it like a PC. Relatively easily, if someone else has done it.
Microcontroller programming
Now let's look at a typical program in Arduino for reading a "10k ohm potentiometer", an extremely simple task that is typical of what you might be doing:
/*
Smoothing
Reads repeatedly from an analog input, calculating a running average
and printing it to the computer. Keeps ten readings in an array and
continually averages them.
The circuit:
* Analog sensor (potentiometer will do) attached to analog input 0
*/
// Define the number of samples to keep track of. The higher the number,
// the more the readings will be smoothed, but the slower the output will
// respond to the input. Using a constant rather than a normal variable lets
// use this value to determine the size of the readings array.
const int numReadings = 10;
int readings[numReadings]; // the readings from the analog input
int readIndex = 0; // the index of the current reading
int total = 0; // the running total
int average = 0; // the average
int inputPin = A0;
void setup() {
// initialize serial communication with computer:
Serial.begin(9600);
// initialize all the readings to 0:
for (int thisReading = 0; thisReading < numReadings; thisReading++) {
readings[thisReading] = 0;
}
}
void loop() {
// subtract the last reading:
total = total - readings[readIndex];
// read from the sensor:
readings[readIndex] = analogRead(inputPin);
// add the reading to the total:
total = total + readings[readIndex];
// advance to the next position in the array:
readIndex = readIndex + 1;
// if we're at the end of the array...
if (readIndex >= numReadings) {
// ...wrap around to the beginning:
readIndex = 0;
}
// calculate the average:
average = total / numReadings;
// send it to the computer as ASCII digits
Serial.println(average);
delay(1); // delay in between reads for stability
}
That's it. On the one hand, it works at an extremely low level, as compared with running desktop applications, connecting to a database, saving a video file, or any number of other tasks. On the other, it is not making use of any complicated programs, frameworks, or languages. The sendmail link I posted that can send to gmail goes through many, many more low-level functions than what I've just quoted. So does Python or any other scripting language you use. The Arduino code is extremely simple, but works line by line at a low level, or uses libraries that are a few KB in size. An installation of just a database like MySQL might be 80+ megabytes on the Raspberry Pi, while the entire memory of an Arduino-compatible board might be 8KB (in the case of the Arduino-compatible Attiny85). That is a difference in size of 10000x (80MB vs 8 KB). You can see someone bump into this wall, writing just a few low-level LED commands.
There are some libraries that enable additional functionality, but the speed of a microcontroller is limited to a few megahertz, it has a limited amount of memory, and it doesn't run a complete operating system like Linux, Windows or a Mac. So why do people use it?
But look at this line, from the above code example:
* Analog sensor (potentiometer will do) attached to analog input 0
That's the magic line. The interface with the real world. Here's how the circuit looks powered up:
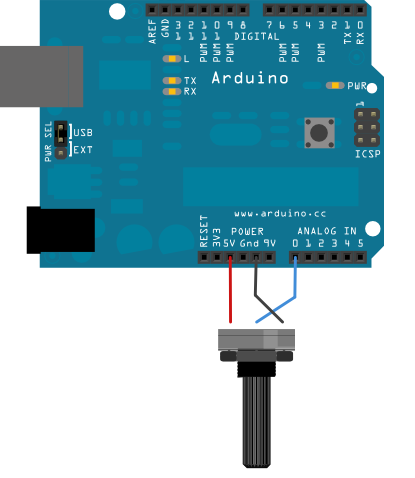
And there is a lot of potential to get things wrong while you do that. But if you don't get it wrong - now you're reading a potentiometer, which is essentially an analog knob you can turn:
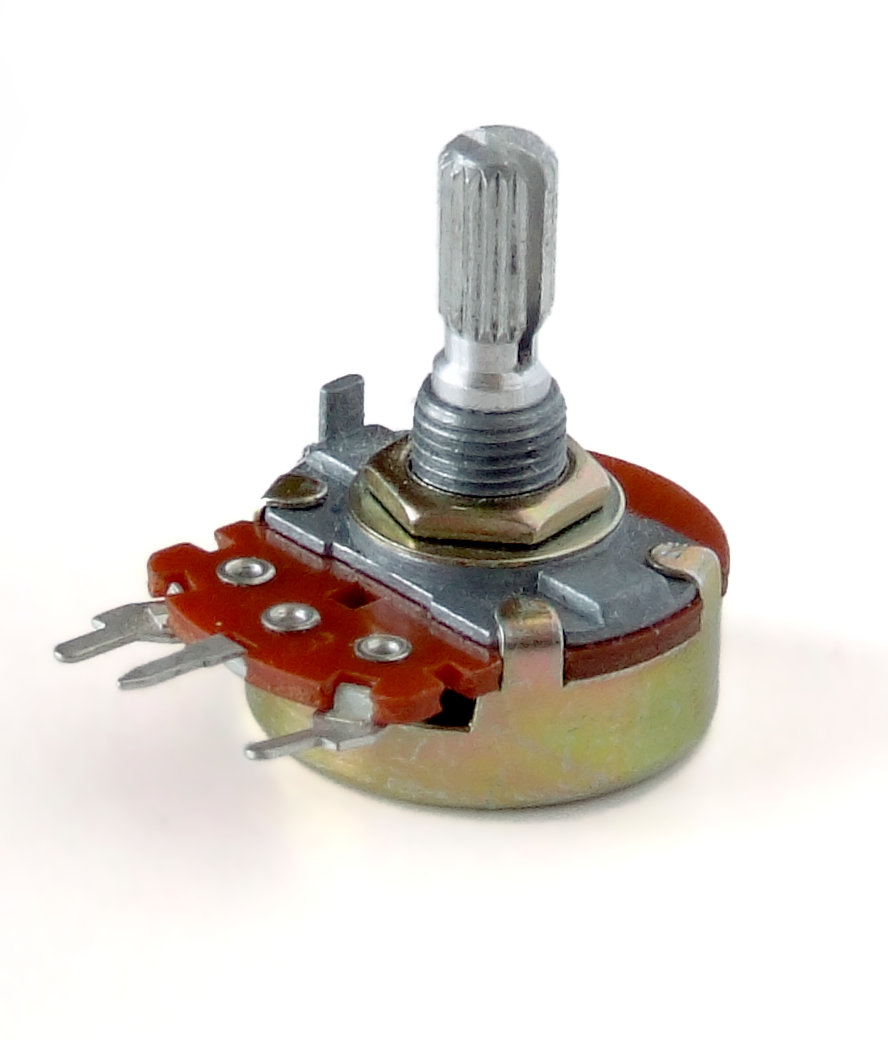
A 10k ohm potentiometer costs $0.25 (okay, up to a dollar or two in a quantity of one). For pennies, you can build an analog dial to your specifications, read it, and drive some motor based on the results. But you will have to come up with the circuit diagram. Which requires a relatively deep level of understanding. For example, why 10k ohm? What even is an ohm? You have to learn these things, get the components, experiment and copy circuits.
Microprocessors are at a lower level, at the level of circuits. They require using a breadboard (which looks like this 

to wire up components in circuits, getting resulst that look like this:

to get servos and motors that look like this:


and maybe even like this:


setting them up to eventually make this

and then if they go into industry as adults, they'll be making

and then if they go into industry as adults, they'll be making
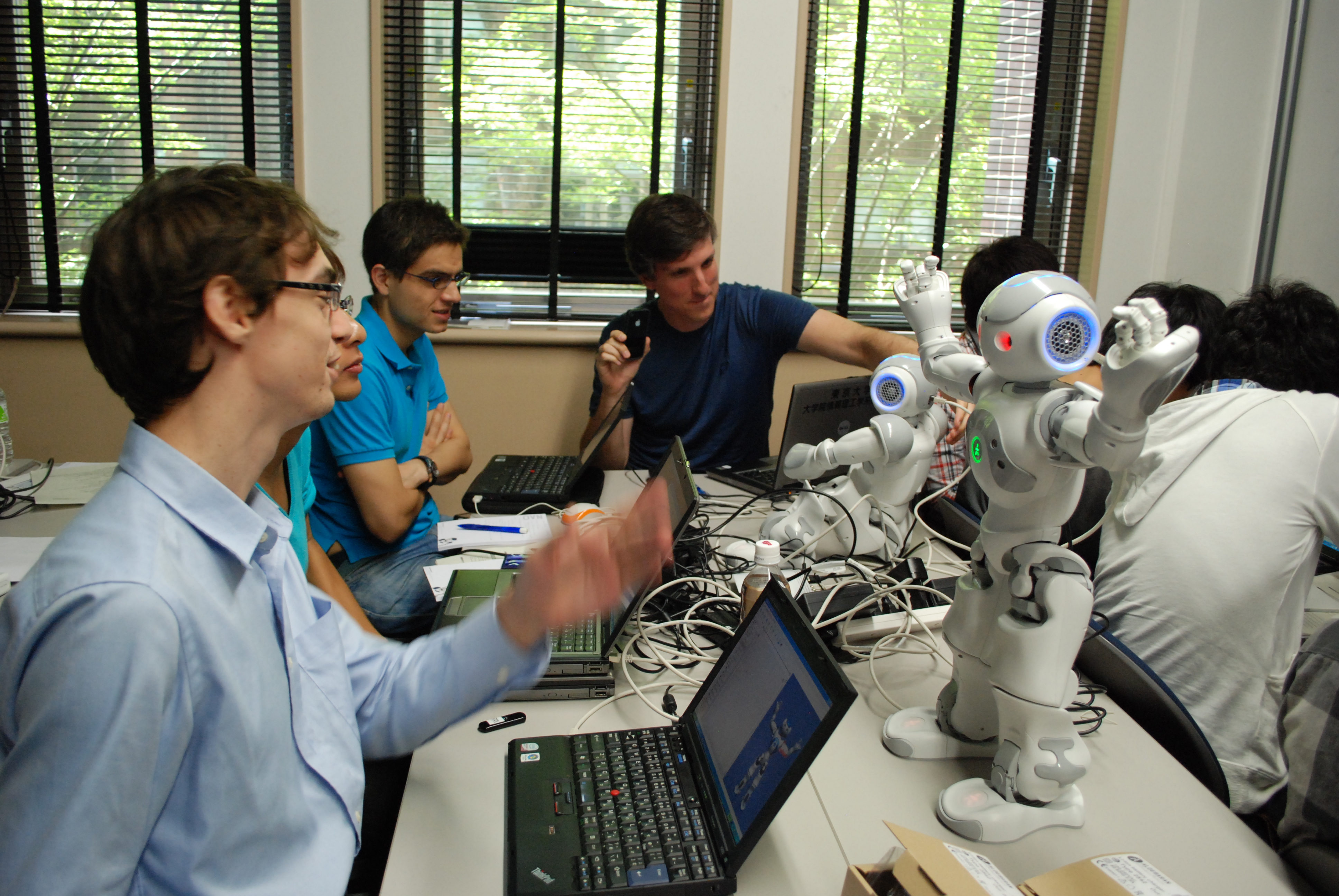
On the PC side, without analog inputs, how would you even get past the very first step - reading a single potentiometer or analog input? I suppose you could find some kind of ready-made dial that interfaces over USB. But this will neve scale or introduce anyone to electronics.
Arduino, and microcontrollers, is about learning and using the things that are interfacing with the real world, from garage openers and thermometer readers, to complex robotics. Of course, just as in the last picture the robot is certainly interfacing with a PC or computer driving it, it is likely that at some point you might again circle back to knowledge of Linux and a PC. But only after you have built and used the circuits that power devices that interact with the real world.
Summary
So the entire nature of the whole endeavor is completely different. I found that moving into the microcontroller things was a steep learning curve, particularly due to the need to wire things up. It's not "plug and play." You have to figure out what's going on, read, and follow new kinds of instructions, specifically, breadboards, components, circuit diagrams. Luckily, the Arduino community is an extremely good resource for getting started.
So, that about sums up what you can expect when moving into Arduino-type electronics from PC's:
1) You will be able to do real-world things, actually build something that moves or interfaces with the analog world we live in. It's not plug and play USB interfaces anymore.
2) You have to learn and understand completely new things, specifically circuits, and programming a Microcontroller in Arduino or another low-level language.
3) Nothing will work the first time. You might destroy some small components.
4) The hardware used are different. You will need a microcontroller, which speaks a low level language like C, called Arduino, or you can program microcontrollers directly in a similar way, and flash these from a PC. You will also be using electronics components.
Eventually, if you want, you can move to programming the same microcontrollers that are used in real-world electronics devices.
At the end of all this, the reward should certainly be worth it. But it is a big transition, to be sure. I hope you've found this post helpful in reviewing some of the things you might expect moving from PC's to Arduino-type Electronics. Of course, electronics goes very deep! After all, even within a PC someone had to design every single resistor or transistor that is on the motherboard of your computer, which would have a circuit diagram that runs into hundreds of thousands of pages. Including the billion transistors in your CPU itself. While you never have to go that far - just as, as a PC user, you will not have to dive into Kernel code on the programming side - the whole nature of working with electronics is different from working with a PC.
At times, it's frustrating, but also a lot of fun. Just don't expect anything to work the first time. Even if you've copied and pasted it.
Welcome to the world of microcontrollers and electronics, and I hope you will learn a lot of new things.



